Policy
- Coding style must be coherent and easy to cross reference. Here as a sample case, will define a loose rule[1][2]at the outset. Referencing these rules, will contribute to understanding evena more difficult code.
- Rules have constructed from experience as well as preference. Should not be too difficult to switch to different rules.
- There may be parts not yet considered or gray portions. Further the rules may not beuniversally targeted[3] Those are the limitations of the rules.
Logical coding language
Using verilog HDL(IEEE1364-1995)
- In logical design, template patterns are limited, hence it is unnecessary to use a high level language
- It is one of the major languages and adaptability to retail tools or inhouse tools is high
- Classical language which has the potential for continuous use in future as well as convertibility to high level language is also easy
- Will consider usage of high level language such as assertion or C for desiging cores
Function・Module Naming
CamelCaserules will be the basis for naming
- First word will be bsically lower case letters, Lower Camel Case
Lower Camel Case → lowerCamelCase
- omitted words to be uniform
Clear Inside Layer → clrInLyr
- Abbreviated usage(search possible)is fine for all capital letters, but first word only to be lower letters
Put Identifier → putID
Identifier Selector → idSel
- Use underscore for negative logic(Must not use negative logic as much as posisble to avoid logical confusion unless there is no alternative due to the specifications of connections at the connector)
reset → reset_n
Connections
To have combination naming revealing the mutual connection of modules from higher levels.
- Delimiter(Underscore)is to be used or not depends on the complexity of mutual connections
- Referring to the below variations, in a single layer, to unify in one of these
- To use the name of the starting module
Output signal of ModuleX → xSig, x_sig
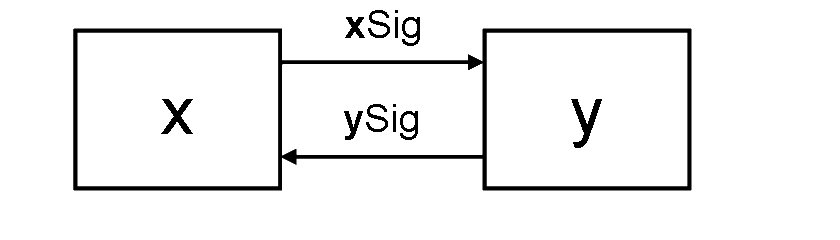
- To use term reflecting both starting and ending module names
Signal from ModuleX to ModuleY → x_ySig
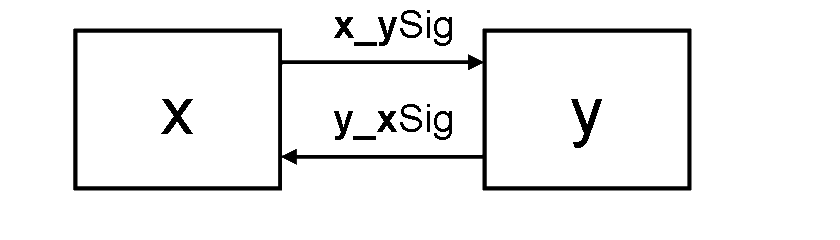
- If there are multiple ending modules then to not explicitly reference the module or to replace with a specific symbol
Signal from ModuleX,W to ModuleY,Z → xSig, w_sig
Signal from ModuleY,Z to ModuleX,W → gSig, g_sig(Replace with g)
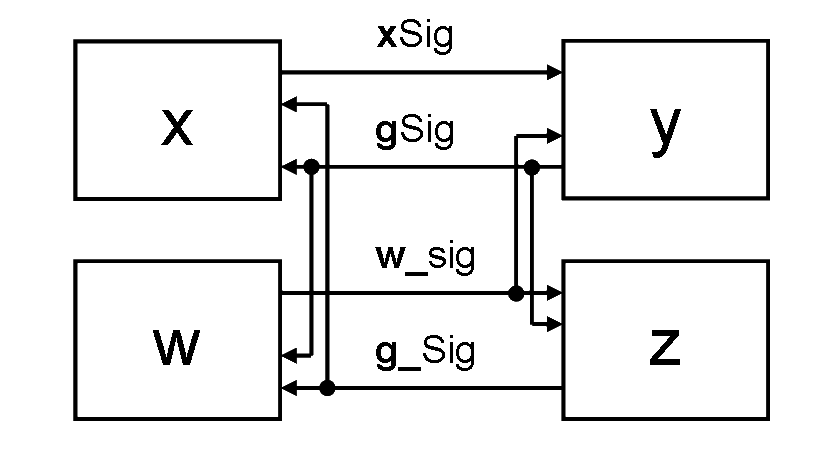
- To use terms coming from the pipeline stage
After pipeline stage A → aSig, a_sig
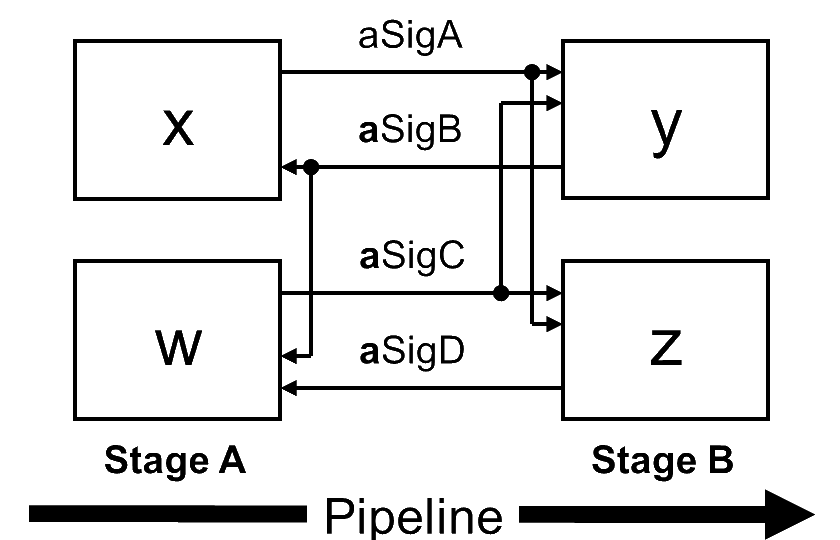
Module
- Connector name to not depend on the module name but rather to be general or conventional
Pipeline input signal → iSig
Pipeline output signal → oSig
Register input signal → regSig
- To use common names like clk or reset throughout
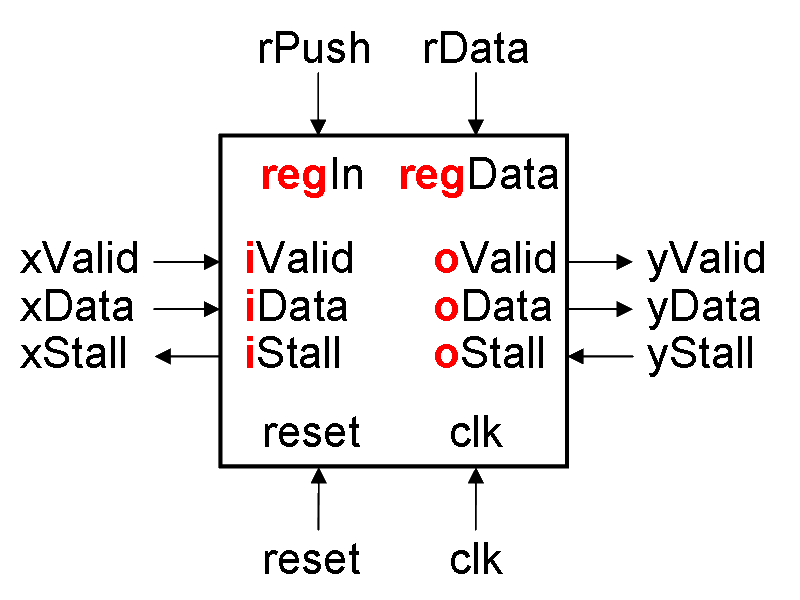
- To use port connections when creating instances
// Instantiation
modX insX (
.iData (wanData),
.iVld (wanVld),
.oData (nynData),
.oVld (nynVld),
.reset (reset),
.clk (clk)
);
- Optimize FF output logical levels, and if the part is at the layout boundary then also to optimize FF pre-input logical levels also.
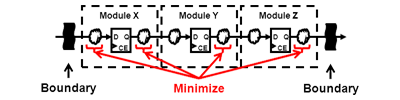
Environmental functions
- If parameters can be used then to use parameters(as it is affected variously by tools)
- Code using Capital letters and use a unique name for highest level module name
// KEY definition with myDMA Module
module myDMA();
...
`define MYDMAKEY 1
...
- When defining with outer core module, to use default definitions
// KEY definition with higher level module than myDMA Module
module myDMA();
...
`ifdef MYDMAKEY
`else
`define MYDMAKEY 1
`endif
Parameter
- Use Capital letter definition for each module
- When overlaps levels, then to use as much as possible common names
module modX(argsX);
...
paramter DATAWIDTH = 32
...
modY #(DATAWIDTH) insY(argsY);
...
endmodule
module modY(argsY);
...
paramter DATAWIDTH = 16
...
modZ #(DATAWIDTH) insZ(argsZ);
...
endmodule
FF coding
- Not defining Instance, to use Non-blocking substitutes (forbid Blocking substitutes)
- Basically to define a procedure for each operation(always procedure)
- Use asynchronous reset depending on condition(Reset circuit)
- To place variable delay so that can visually check using wave type viewer(Changes slightly delayed from clock edge)
// FF Description (Normal)
reg oVld;
always @(posedge clk or negedge reset_n)
if (!reset_n) // Asynchronous Reset
oVld <= #1 1'b0; // Fixed Delay #1
else if (reset) // Synchronous Reset
oVld <= #1 1'b0;
else if (!iStall) // Enable
oVld <= #1 iVld;
- If D type FF conding is required(State Machine coding)、to use variables with D attached to the end
// FF Description (D Type FF)
reg oVld;
wire oVldD;
always @(posedge clk or negedge reset_n)
if (!reset_n)
oVld <= #1 1'b0; // Reset Port
else
oVld <= #1 oVldD; // Data Port
assign oVldD = reset ? 1'b0 : iStall ? oVld : iVld;
Combination circuits coding
- To use continuous substitution using "assign" command or blocking substitution using "always" command
- For Blocking substitutes to use as much as possible default values at start of substitution(Especially when "case" command is used for complex condition operation)
- When using "function" command, to take care that local variables do not overlap(to handover all values at input)
// Blocking (assign oVldD = reset ? 1'b0 : iStall ? oVld : iVld)
reg oVldD;
always @(reset or oVld or iVld or iStall) begin
oVldD = oVld // Default set prevents from latch gen
if (reset) // Synchronous Reset
oVldD = 1'b0;
else if (!iStall) // Enable
oVldD = iVld;
// else // Necessary if not default set
// oVldD = iVld;
end
Other than above
- As much as possible to code keeping circuit high speed optimization in mind
// Selector
wire [31:0] dataA, dataB, dataC;
wire selA, selB, selC;
wire [31:0] selOut;
...
// assign selOut = selA ? dataA : selB ? dataB : dataC;
assign selOut = {32{selA}} & dataA
| {32{selB}} & dataB
| {32{selC}} & dataC;
...
- Tool dependent coding to avoid as mush as possible(For example "full_case" or "parallel_case" of synopsis)
Circuit Design > Home > Language・Coding Style Next Page(Protocol used) TOP of this page ▲